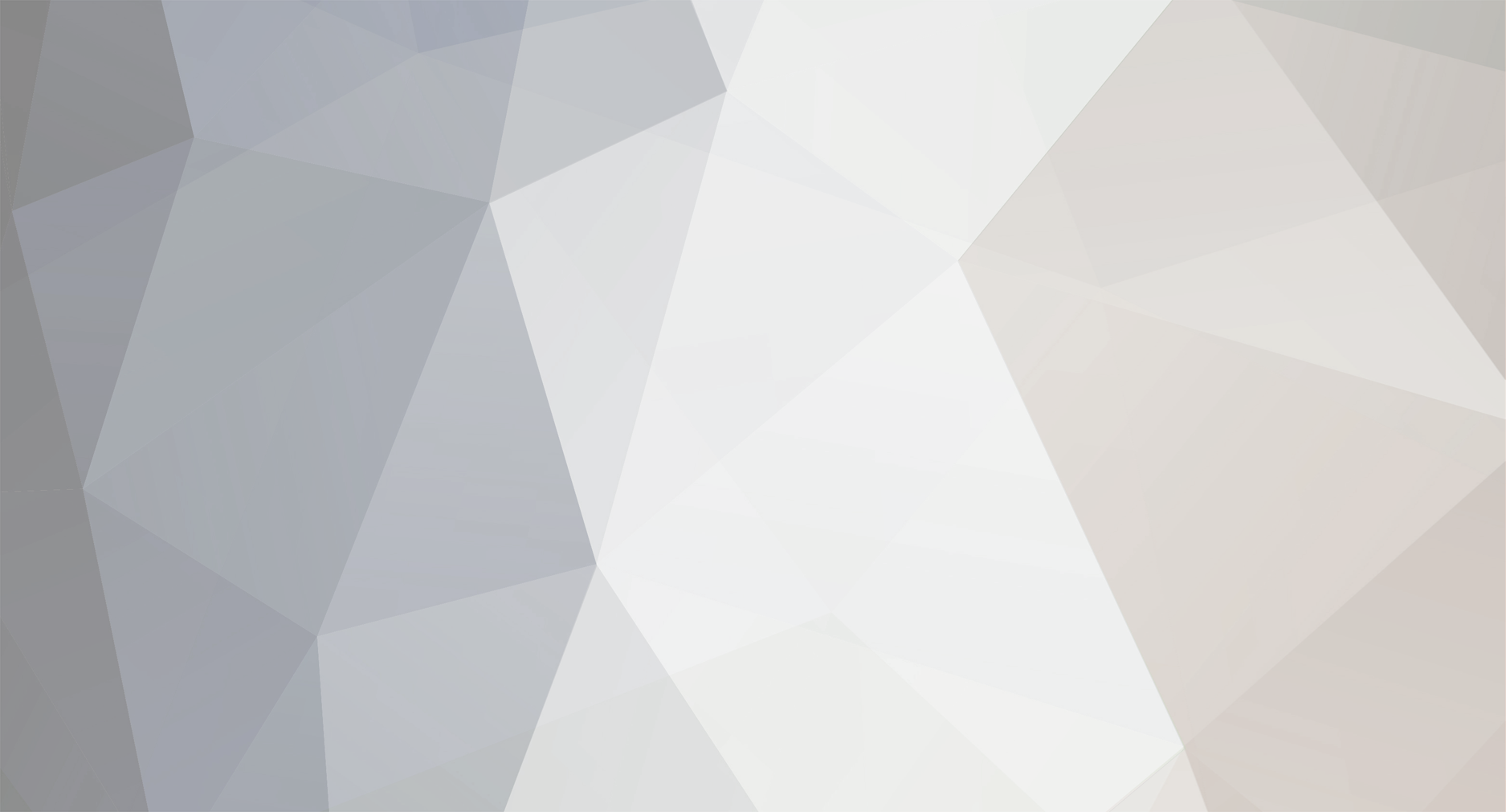
Ideka
-
Posts
38 -
Joined
-
Last visited
-
Days Won
6
Content Type
Profiles
Forums
Downloads
Calendar
Bug Tracker
Posts posted by Ideka
-
-
My latest accomplishment in ASM hacking:
Coded the actor in pure MIPS assembly. I suppose it was a bit trickier than it'd have been with C, but it allowed for a very optimized overlay at only 0x310 bytes long.
-
1
-
-
I might be wrong, but couldn't it just be a slightly modified image of two normal disks? It's weird that this crappy photo was the only one this dude managed to get up with that long story.
-
Okay. The last month(s?) I've been taking a break from the whole hacking thing, simply because I didn't have any time to spare for it. But now that Summer's coming up, I can gradually start picking up this hobby again! Here's a code I wrote quickly for a simple "marathon" mini-game where you gain 200 rupees if you win, or lose them if you don't:
.org HOOK J game nop .org 80600000 game: Lui t0, UPPER ADRESS OF OPPONENT Z POSTION Lh k0, LOWER ADRESS OF OPPONENT Z POSITION(t0) ; loads the opponent z coordinate into k0 Li k1, c58f ; Z coordinate for winning point Beq k1, k0 lose ; if the opponent's z postition is equal to the winning point, branch to lose nop Lui gp, 8022 Lh gp, 45dc(gp) ; loads link's z coordinate into gp Beq k1, gp, win ; if link's z position is equal to the winning point, branch to win Nop j game nop Win: Sw r0, LOWER ADRESS OF OPPONENT Z POSITION(t0) ; sets the opponent's z position to 0 Lui t0, 8016 Lh t1, e694(t0) ; loads rupee amount Addiu t1, t1, 00c8 ; calculates new rupee amount Sh t1, e694(t0) ; stores new rupee amount J return: Lose: Lui t0, 8016 Lh t1, e694(t0) ; loads rupee amount Addiu t1, t1, fe00 ; calculates new rupee amount Sh t1, e694(t0) ; stores new rupee amount Return: J game ; jumps back to start of hack Nop
I obviously need to locate the adress of the Z coordinate of the opponent actor (which I plan to be the Running Man), but this is quite easy. My major problem is finding a good hook that runs every frame. If any of you guys have any tips or feedback on this hack, please tell me! Anything would be appreciated, really. Thanks.
-
-
Hmm... Using ANDI doesn't seem to work very well, it always makes the effect present with multiple other tunics. Perhaps I'm using it the wrong way? Here's the most recent source (haven't looked into the SLTI opcode yet):
.org 80087234 j 80600000 nop .org 80600000 lui k0, 8016 lb k1, e6d0(k0) andi k1, k1, 0003 bnel k1, r0, magic lb k1, e693(k0) j return sh t9, 0030(t0) magic: bgezl s0, return sh t9, 0030(t0) blezl k1, return sh t9, 0030(t0) li t2, 0004 beql t2, k1, return sb r0, e693(k0) li t2, 0003 beql t2, k1, return sb r0, e693(k0) li t2, 0002 beql t2, k1, return sb r0, e693(k0) li t2, 0001 beql t2, k1, return sb r0, e693(k0) addiu k1, k1, fffa j return sb k1, e693(k0) return: j 80087238 lh a1, 0030(t0)
Thanks for any help!
-
Wow, thanks a lot Jason, I'm impressed by how easily you analyzed my hack! Those are definitely really useful advice, I think my opcode list is quite outdated (circa 98) so I'll look into that to avoid future mistakes. And yes, I have it patched to ROM. I would release a patch, but there's a possibility that this is going to be used in a major hack, so I'll wait until that's decided.
-
So, I've devoted a few weeks to it, and I think that I'm finally starting to get a grasp around the basics of ASM hacking. Here's where I'll post any future major hacks I'll eventually make.
OoT Magic Armor:
Source:
.org 80087234 j 80600000 ; jump to routine nop .org 80600000 lui k0, 8016 ; load upper adress into k0 lb k1, e6d0(k0) ; load current equipped tunic value into k1 li t2, 0013 ; load 0013 (normal boots and zora tunic equipeed value) into t2 beql k1, t2, magic ; if k1 is equal to t2, branch to "magic" label lb k1, e693(k0) ; delay slot, loads current magic value into k1 if above statement is true li t2, 0023 ; load 0033 (heavy boots and zora tunic equipped value) into t2 beql k1, t2, magic ; if k1 is equal to t2, branch to "magic" label lb k1, e693(k0) ; delay slot, loads current magic value into k1 if above statement is true li t2, 0033 ; load 0033 (heavy boots and zora tunic equipped value) into t2 beql k1, t2, magic ; if k1 is equal to t2, branch to "magic" label lb k1, e693(k0) ; delay slot, loads current magic value into k1 if above statement is true j return ; if any of the above statements are false, jump to "return" label sh t9, 0030(t0) magic: bgezl s0, return ; if new health value is greater than zero, branch to "return" label sh t9, 0030(t0) ; blezl k1, return ; if magic amount is less than or equal to zero, branch to "return" label sh t9, 0030(t0) li t2, 0004 ; load 0004 into t2 beql t2, k1, return ; if k1 is equal to t2, branch to "return" label sb r0, e693(k0) ; delay slot, nulls the magic amount if above statement is true li t2, 0003 ; load 0003 into t2 beql t2, k1, return ; if k1 is equal to t2, branch to "return" label sb r0, e693(k0) ; delay slot, nulls the magic amount if above statement is true li t2, 0002 ; load 0002 into t2 beql t2, k1, return ; if k1 is equal to t2, branch to "return" label sb r0, e693(k0) ; delay slot, nulls the magic amount if above statement is true li t2, 0001 ; load 0001 into t2 beql t2, k1, return ; if k1 is equal to t2, branch to "return" label sb r0, e693(k0) ; delay slot, nulls the magic amount if above statement is true addiu k1, k1, fffa ; decreasing the current magic amount stored in k1 j return ; jumps to "return" label sb k1, e693(k0) ; stores new magic value into k0 return: j 80087238 ; jumps back to original routine lh a1, 0030(t0)
Yes, it could probably be made a lot shorter, but there's nothing I can do with my current capabilites.
Thanks for reading, and keep a look out for any future updates!
-
11
-
-
Slightly off-topic, but why did you name this topic exactly like SoD's old topic(if I remember it correctly) over at glitchkill? Also, like haddockd said, it would be a good idea to actually mention what you are having issues with.
-
Wow, thanks so much everybody! I didn't expect to get these many replies, it's very appreciated. I'll look into all of these things soon. I had heard that $801A0000 was freespace, but I'll be sure to try with $80600000 as well. Also I got the Renegade assembler working, which should help me greatly. Again, thanks!
-
Hey everyone!Recently I decided to finally get my ass off and take on the huge task of learning r4300i disassembly. I have basically spent the last two weeks just trying to find documentation on the subject, and learn whatever I could from it. This, I hope anyways, has probably given me some decent knowledge in the very basics of MIPS disassembly.A few days ago I started trying to write ASM hacks of my own (for the OoT MQ debug ROM). Obviously I wanted to start with something simple, namely that the current Rupee amount would increase by 1 each time that the D-pad up button was pressed. I used the source ofas a base when (trying) to write the hack, which resulted in what I thought was a pretty legit-looking code. Of course it didn't work at all in-game. The closest result I managed to get was that the game paused each time that D-pad up was pressed, which is kinda cool but not very close to the desired effect.I have tried to fix the problem by myself for a time now, but I just don't seem to have the knowledge to locate the problem. I suspect that some instruction might be missing, or that I may have constructed the hack in a weird way. Another, "sillier" possibility is that I've just assembled the gs code incorrectly, since I had to do it manually due to neither the Renegade64 nor Galatea assemblers working correctly on my computer. I haven't been able to find any of these issues though, and I have checked the gameshark code multiple times for faults I couldn't find. I think that the solution simply just lies beyond me and my current capabilities.I hope that maybe some of our experienced and knowledgeable MIPS-disassemblers will show up and save the day, but any help would be mostly appreciated really. Anyway, here's the source and gs code:
Source:
.ORG 0x80047E50 J 0x801A0000 NOP .ORG 0x801A0000 LUI T0, 0x8016 ; Loads upper adress to rupee amount in T0 LH T4, 0xE694(T0) ; Loads halfword from T0 into T4 LUI T0, 0x8016 ; Loads upper adress to controller buttons LHU T2, 0x6AF0(T0) ; Loads unsigned half-word into T2 from T0(controller buttons) ADDIU T3, R0, 0x0800 ; Dpad-up stored into T3 BEQL T2, T3, 0x801A001C ; If T2(controller buttons) equals to T3(Dpad-up pressed), branch to 0x811A001C ADDIU T4, T4, 0x0001 ; Adds immediate value 0x0001 to T4(current rupee amount) .ORG 0x801A001C LUI T0, 8016 ; Loads upper adress to rupee amount SH T4, 0xE694(T0) ; Stores new rupee amount(T4) into current rupee amount(T0) J 0x80047e58 LH V0, 0xE690(V0) ; "this is what was replaced at 0x80047E50" (source: savestate) SLTI AT, V0, 0x0011 ; "if taken out it would cause a lot of trouble" (source: savestate) NOTES: 0x8015E694 = Rupee amount
GS code (assembled by hand, may be incorrect):81047E50 0806 81047E52 8000 81047E54 0000 81047E56 0000 811A0000 3C08 811A0002 8016 811A0004 850C 811A0006 E694 811A0008 3C08 811A000A 8016 811A000C 950A 811A000E 6AF0 811A0010 240B 811A0012 0800 811A0014 516A 811A0016 8006 811A0018 258C 811A001A 0001 811A001C 3C08 811A001E 8016 811A0020 A50C 811A0022 E694 811A0034 0801 811A0036 1F96 811A0038 8442 811A003A E690 811A003C 2841 811A003E 0011
And again: any help would really mean a lot to me! Thanks for reading! -
I can help you with this one, just shoot me a message on Skype.
-
Today is the day, huh? Awesome work, I'm very impressed by how fast you got this done.
-
1
-
-
That is because I converted the model with a tool that isn't hardware compatible. I could try to fix it if you want, but I would have to do it later in the week since I'm quite busy now.
-
I've only been playing for like 5 minutes when this is being posted, so far most things seems fine except for three minor issues:
1. The game freezes when trying to open the menu
2. Many sentences seems to be missing punctuation
3. The cutscene disabler still seems to be functional
-
I sure will be playing this one B-) Isn't this like the first full zoldo hack in 2 years(since Voyager of Time)? Awesome work on getting it completed, now we'll just have to bring attention to it.
I suggest (if you haven't already done it) posting threads about this in multiple forums that may have interest in it, like Zelda Universe, Zelda Speedruns, Zelda Dungeon etc. And again: awesome work on actually getting this project done. That seems to have been a rare quality among Zelda modders through the years.
-
The triforce shards most likely won't work on hardware. I haven't had time to figure out how Model2F3DEX2 works yet, so I just used Model2N64 to convert the model. Also, didn't you say that you didn't really care for hardware-compability? Just tell me if you want me to try and re-doing them.
-
Can't remember where I found this, but I think it was made by Flotonic:
FD Command:
Format:FD TT 00 00 BB XX XX XXTT = Texture TypeBB = Texture BankXX XX XX = Location of Texture in whatever the bank isDescription:It makes your model look pretty with textures!Example:You find this in a Display List:FD 48 00 00 04 01 17 80Compare to format:FD TT 00 00 BB XX XX XXYou're probably like "OHH MY GOSH!!!! BB IS 04 AND I DON'T KNOW WHAT THAT IS!" Smart people refer to the BANK LISTING. 04 signals that the texture is located in and loaded from gameplay_keep. You'd change the bank to 06 if you're changing it to use a texture already in the .zobj, obviously, and you'll be changing the location. Anyway. From this, we can tell that the texture type is 48, an 8-Bit CI texture. It's located at 0x11780 in gameplay_keep. -
Now THAT is something I surely will be following! Finally we may get a possible full hack for MM.
-
Alright, I'll PM you some pics soon. Also, have you heard about Flotonic's MM to OoT map porter? Works flawlessly as far as I can tell, aside from not porting the actors (which pretty much would be impossible anyway, since MM has many actors that doesn't exist in OoT).
-
I do have some discontinued mini-dungeon buried in some ancient folders. I'm not sure how pretty it is though, and my mapping skills at that time were quite horrible. If I remember things correctly, the theme of the map was a museum break-in... Not sure how well that would fit...
-
Sure, I could import some of them for you. Do you have Skype or anything? It makes direct file sharing quite simple
-
I'm happy to know that I contributed something to this mod, even if it was in-direct
. Can't wait to play the final product, looks amazing so far!
-
I'm planning to use this for some mini-mod in the future, don't get your expectations up though. Not quite sure what more to say about it...
-
6
-
-
I guess by small, I meant in relation to the size of the head display list, but here it is (starts at offset 0x9298 in object_gnd.ZOBJ):
E7 00 00 00 00 00 00 00 E3 00 10 01 00 00 00 00 D7 00 00 02 FF FF FF FF FD 10 00 00 06 00 BE 80 F5 10 00 00 07 0D 00 40 E6 00 00 00 00 00 00 00 F3 00 00 00 07 0F F2 00 E7 00 00 00 00 00 00 00 F5 10 08 00 00 0D 00 40 F2 00 00 00 00 03 C0 3C FC 12 7E 03 FF FF F3 F8 E2 00 00 1C C8 11 20 78 D9 F3 FF FF 00 00 00 00 D9 FF FF FF 00 03 04 00 FA 00 00 00 FF FF FF FF DE 00 00 00 08 00 00 00 01 02 00 40 06 00 72 C8 06 00 02 04 00 02 00 06 06 08 00 0A 00 00 08 0C 06 02 0E 04 00 0E 02 0C 06 0E 08 04 00 08 0E 0C 06 10 12 14 00 16 14 12 06 16 12 18 00 10 18 12 06 10 14 18 00 14 16 18 06 1A 1C 1E 00 1C 1A 20 06 22 1C 20 00 1C 22 1E 06 22 1A 1E 00 22 20 1A 06 24 26 28 00 26 24 2A 06 2C 24 28 00 24 2C 2A 06 26 2E 28 00 2E 26 2A 06 2E 2C 28 00 2C 2E 2A 06 30 32 34 00 36 34 32 06 38 34 3A 00 36 3A 34 06 30 3C 32 00 36 32 3C 06 30 3A 3C 00 36 3C 3A 01 00 60 0C 06 00 74 B8 06 00 02 04 00 02 06 04 06 02 08 06 00 04 0A 00 06 00 0A 08 00 08 0A 06 E7 00 00 00 00 00 00 00 FD 10 00 00 06 00 B3 80 F5 10 00 00 07 0D 43 40 E6 00 00 00 00 00 00 00 F3 00 00 00 07 1F F2 00 E7 00 00 00 00 00 00 00 F5 10 08 00 00 0D 43 40 F2 00 00 00 00 03 C0 7C FC 11 FF FF FF FF F2 38 E2 00 00 1C C8 11 30 78 D9 FD FF FF 00 00 00 00 DE 00 00 00 08 00 00 00 01 00 A0 14 06 00 75 18 06 00 02 04 00 02 00 06 06 08 0A 0C 00 0A 08 0E 06 02 10 04 00 10 02 06 06 0A 12 0C 00 12 0A 0E DF 00 00 00 00 00 00 00
How I port the textures:
1. I first look at what offset the FD command points to for the texture
2. Then I use Flotonic's tool "textdoc.EXE" to find the size of the texture(s) I want to port
3. Then I paste them at new offsets, which I write down
4, When I'm done with that I simply repoint the 3 last bytes of the FD commands to their new texture offsets
Thanks.
------------------------------------------
EDIT:
Fixed the problem by removing the DE commands in the display list.
Ideka's ASM hacks
in Modifications
Posted
Like stated in the video description (on Youtube though, so I get why you missed it) that's simply caused by my crappy computer. This was the best, least laggy footage I could manage to record, believe it or not.